
Step 2 of 3: Implementation
In Java, threads can be customized by the programmer in two ways.
-
Extending the Thread class for Java API and overriding the run() :
The Thread Class has a run method which does nothing and has to be overridden by the programmer for performing
their actions.
-
Implementing the Runnable interface:
The second method is to implement the interface "Runnable" :
The Runnable object provides the run() method, which the programmer can implement for their actions.
The choice between these two method are simply based on the restriction of Multiple Inheritance in Java.
-
If your Class which is to implement the Threads run() method has to inherit from some other class (like the Applet
class or any other), then the second method is suitable, as Java does not allow multiple inheritance.
-
Else use the first method of sub-classing the Thread Class.
The Start() method:
The start() method does the following
-
provides the necessary System resources to run the thread,
-
Scheduling the threads to run,
-
and Calling the run() method. Once the thread's start method is executed the thread is in the Runnable mode.
Priority among threads :
-
The execution of multiple threads on a single CPU, in some order, is called scheduling. The Java runtime
supports a very simple, deterministic scheduling algorithm known as "Fixed Priority Scheduling".
-
In Java, threads have priority ranging from MIN_PRIORITY to MAX_PRIORITY, which are constants defined in the Thread
class. Also the priorities can be set using the setPriority() method in the Thread class. At any given time, when
multiple threads are ready to be executed, the thread with the highest priority is chosen for execution.
-
It is important to note that Java by itself does not do Time-slicing, i.e. it will not preempt the current running
thread for another thread of same priority. However at system level, it may implement the time-slicing for Java
threads, depending upon the various systems. It is not good practice to write code, which depends on system
implementation of time-slicing.
-
Finally, a thread can give the green-light, to another thread to execute using the yield() method.
However, the thread to which the given thread yields, should be of same
priority, otherwise it will be ignored.
Stopping or Thread death :
A thread arranges its own death by the use of some terminating condition, like end of iteration, match of some waiting
condition etc. The Thread class provides the isAlive() method, which returns true, if the thread has been started
and is not stopped, and returns false, if the thread is just a new Thread or is dead.
-If true, then it cannot be differentiated between the Runnable and Not Runnable state. -Similarly,
when false is returned, it is difficult to differentiate between a new or dead thread.
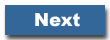
|